Chapter Two - Molding the Memory: From Data Storage to iCloud Sync
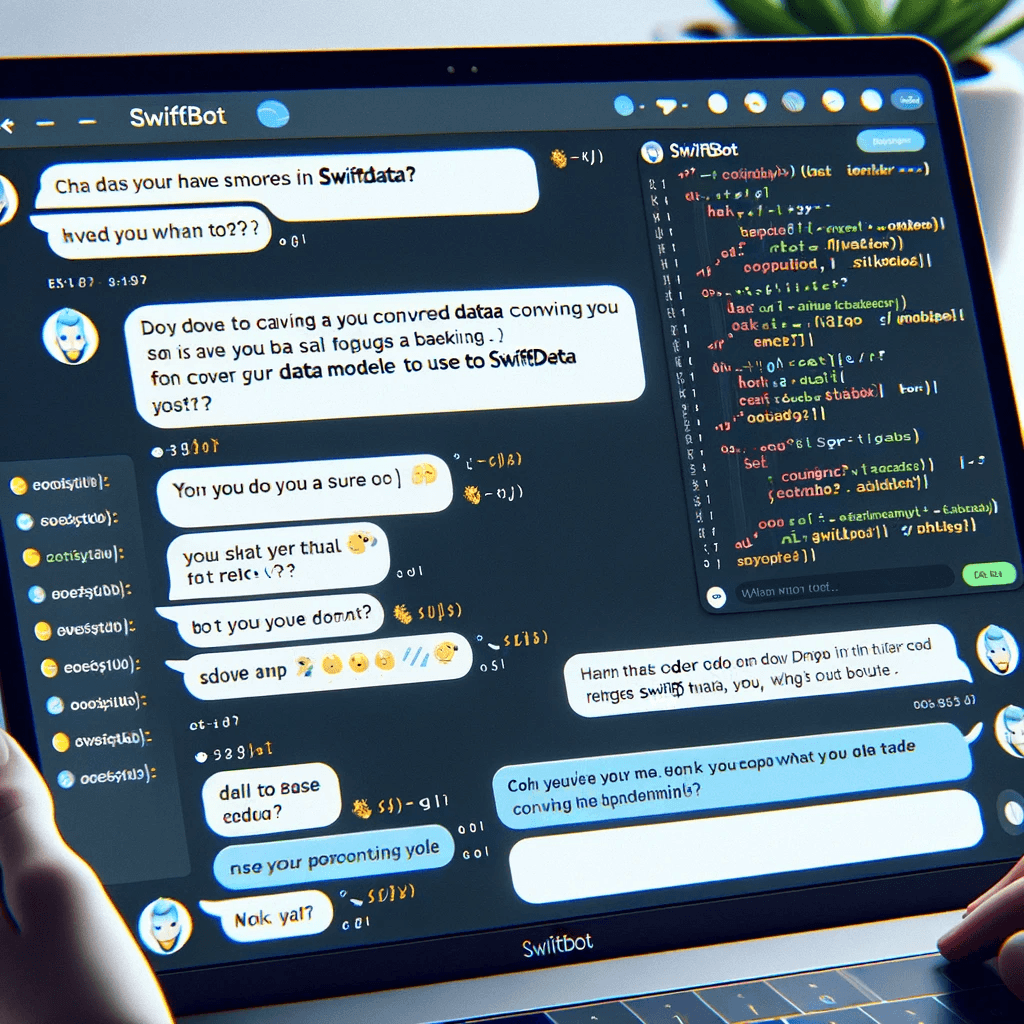
If you thought remembering your second cousin's name was tough, try transitioning an app's data storage system. Welcome to the second installment of our blog series on developing Halfbrain, an AI-powered iOS app for those with "terrible memories." In this episode, we'll dive into how we evolved our data handling by switching to Swift Data and bolstered our app's security with iCloud backups using CloudKit.
Migrating to Swift Data
Our journey began with the built-in data store as showcased in Apple's App Dev Training tutorial. But as our needs grew, so did our ambition. We decided to upgrade to Swift Data for more robust and flexible data management.
The Swiftbot Chronicles
Enter Swiftbot, our custom ChatGPT, loaded with the latest Apple Dev talks on Swift. Here's how it went down:
- Model Migration: I copy-pasted each model from the tutorial into Swiftbot.
- Swiftbot's Magic: Swiftbot then performed its AI wizardry, converting each model into Swift Data-compatible formats.
- Integration and Testing: These models were then integrated back into Halfbrain, followed by rigorous testing to ensure everything was hunky-dory.
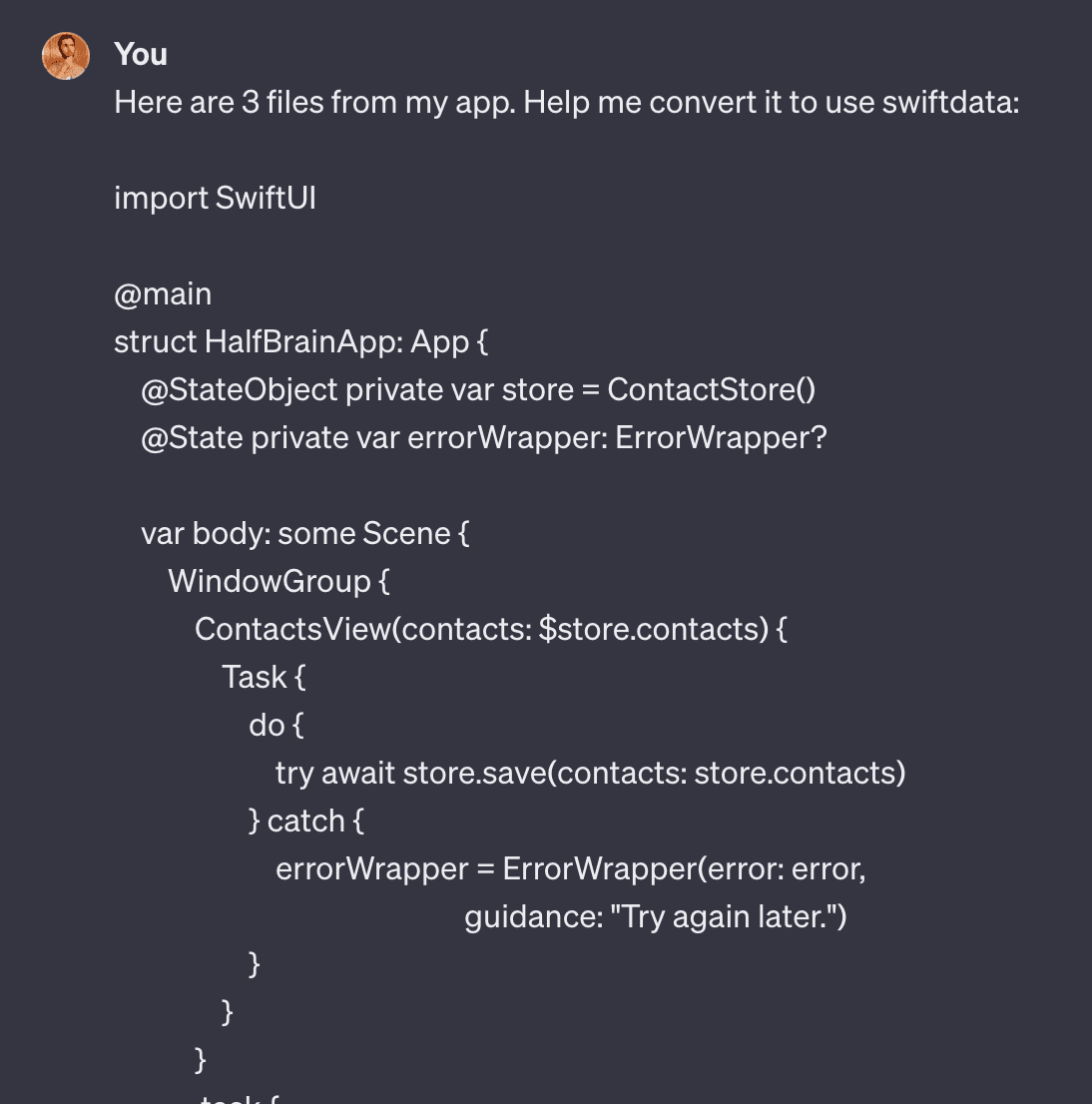
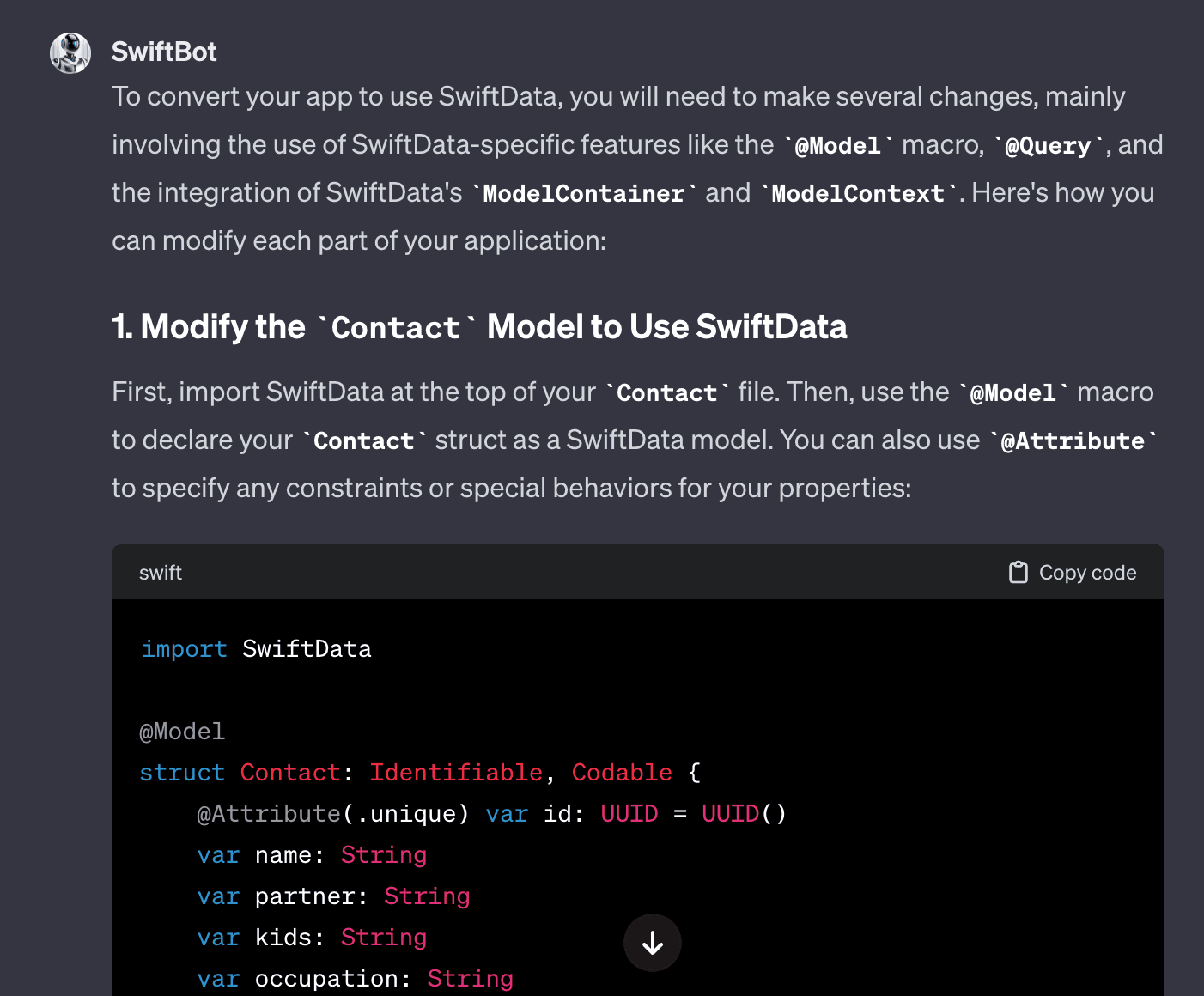
Why Swift Data?
- Performance: Like a sports car compared to a family sedan, Swift Data just zips along.
- Flexibility: It's like having a Swiss Army knife for data – versatile and ready for anything.
Implementing iCloud Backups with CloudKit
Data loss is a nightmare, especially for an app designed to manage memories. So, we turned to iCloud backups via CloudKit.
Swiftbot to the Rescue, Again
Swiftbot was not just a one-trick pony. It also assisted in integrating CloudKit into Halfbrain.
- Guidance from Swiftbot: I asked Swiftbot about implementing CloudKit, and it provided a step-by-step guide.
- Coding Time: With Swiftbot's wisdom, I was able to integrate CloudKit, ensuring seamless data synchronization with iCloud.
CloudKit: Our Data Safety Net
- Ubiquitous Access: Data is now accessible across all devices, like having your memories travel with you.
- Security: Like a vault for your data, keeping it safe and sound.
Glorious Screenshots and Code
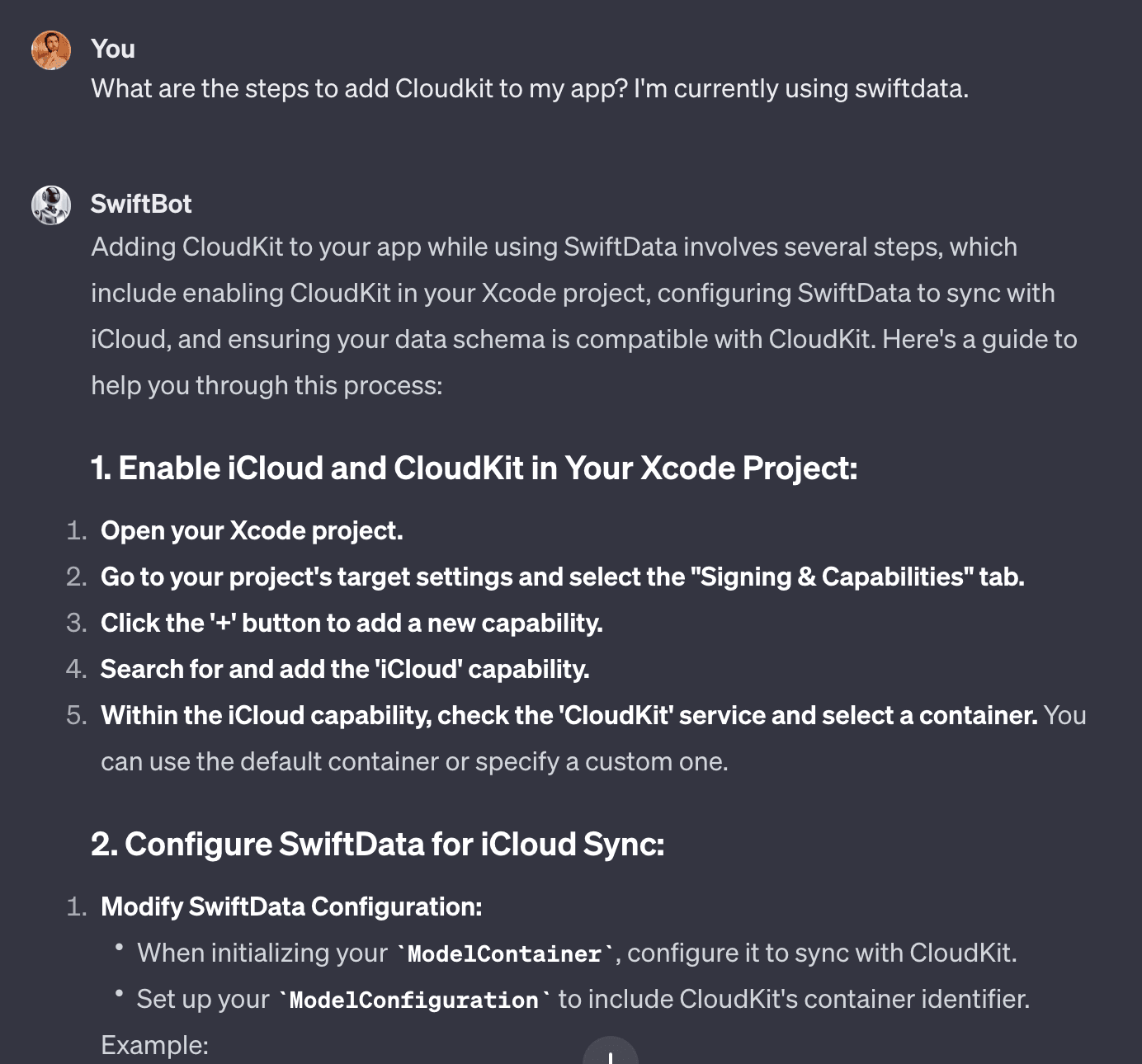
let cloudKitConfig = ModelConfiguration(cloudKitDatabase: "iCloud.com.example.myapp")
let modelContainer = try ModelContainer(for: [MyModel.self], configurations: cloudKitConfig)
import Foundation
import SwiftData
typealias Contact = ContactSchemaV3.Contact
// ... [Include ContactSchemaV1 and ContactSchemaV2 definitions as they are]
enum ContactSchemaV3: VersionedSchema {
static var versionIdentifier = Schema.Version(1, 0, 2)
static var models: [any PersistentModel.Type] {
[Contact.self]
}
@Model
final class Contact {
@Attribute(.unique) var id: UUID = UUID()
var createdAt: Date? = Date()
var updatedAt: Date? = Date()
var name: String = ""
var phoneNumber: String? = nil
var email: String? = nil
var partner: String = ""
var kids: String = ""
var occupation: String = ""
var interests: String = ""
var notes: String = ""
var questionsToAsk: String = ""
var theme: Theme = .sky
var history: [History] = []
// Updated initializer with default values
init(id: UUID = UUID(), createdAt: Date = Date(), updatedAt: Date = Date(), name: String = "", phoneNumber: String? = nil, email: String? = nil, partner: String = "", kids: String = "", occupation: String = "", interests: String = "", notes: String = "", questionsToAsk: String = "", theme: Theme = .sky) {
self.id = id
self.createdAt = createdAt
self.updatedAt = updatedAt
self.name = name
self.phoneNumber = phoneNumber
self.email = email
self.partner = partner
self.kids = kids
self.occupation = occupation
self.interests = interests
self.notes = notes
self.questionsToAsk = questionsToAsk
self.theme = theme
}
}
}
// ... [Include ContactData, extension Contact with Attendee, and other extensions as they are]
Stay Tuned...
So, there you have it - another chapter in the HalfBrain development diary. If you've ever felt like your brain needed a backup drive, stay tuned. HalfBrain.ai is gearing up to be just that. More tales of development adventures (and misadventures) coming soon!
Next time, I’ll dive into the nitty-gritty of securing user data and setting up a subscription model. Because, let's face it, even memory superheroes need to keep the lights on.